PHP is a powerful tool for web development. It’s widely used and easy to learn. If you’re new to PHP, don’t worry. Here are some essential tips and tricks to help you get started.
Start Simple
Begin with the basics. Learn how to set up a PHP environment on your computer. You can use tools like XAMPP or MAMP. These tools make it easy to run PHP locally.
Understand Syntax
PHP syntax is similar to C and Java. Practice writing simple PHP scripts. For example, try creating a script that displays “Hello, World!” on your browser. This will help you understand how PHP works.
<?php
echo "Hello, World!";
?>
Variables and Data Types
Learn about variables in PHP. They are used to store data. PHP supports different data types like strings, integers, and arrays. Here’s how you declare a variable:
<?php
$greeting = "Hello, World!";
echo $greeting;
?>
Use Comments
Comments help you understand your code later. Use //
for single-line comments and /* */
for multi-line comments. Good comments explain what your code does.
<?php
// This is a single-line comment
/* This is a
multi-line comment */
?>
Control Structures
Master control structures like if
, else
, while
, and for
. They control the flow of your program. For example:
<?php
$number = 10;
if ($number > 5) {
echo "Number is greater than 5";
} else {
echo "Number is 5 or less";
}
?>
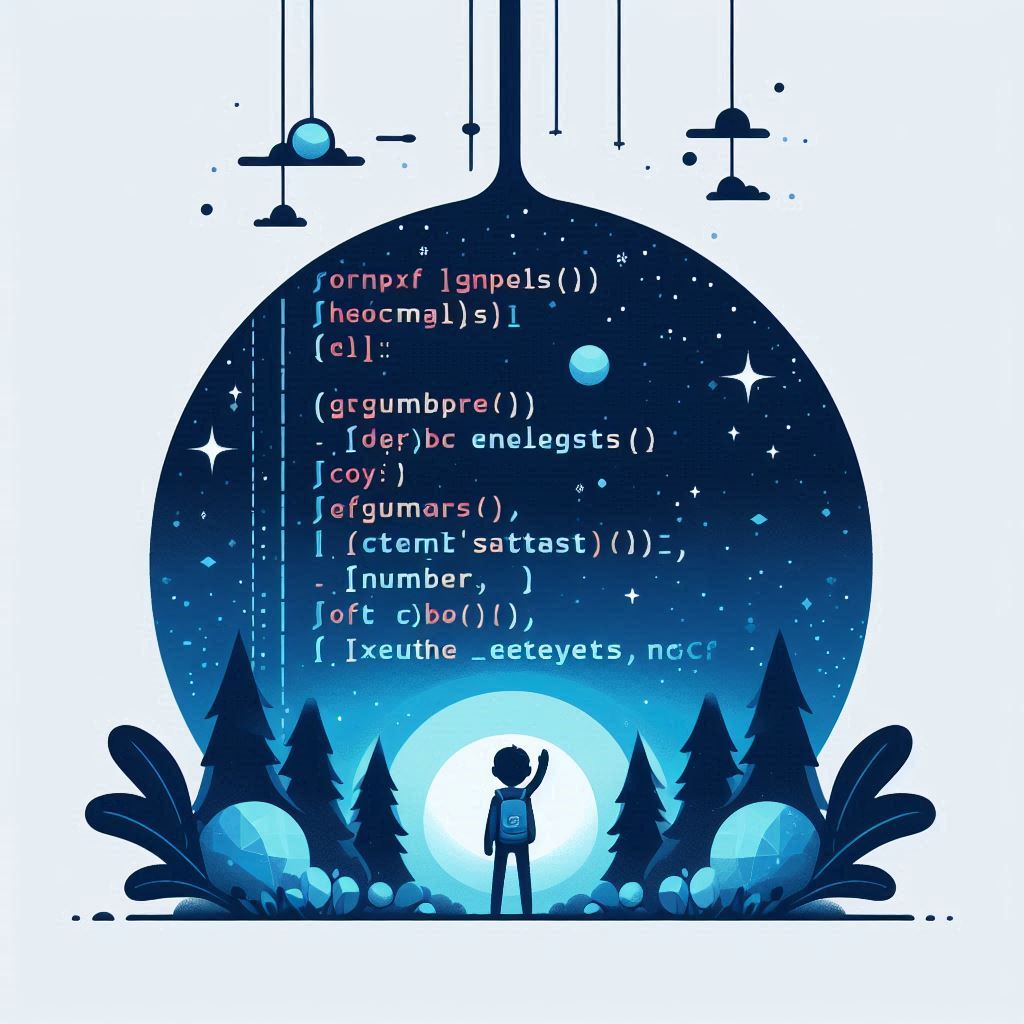
Functions
Functions are reusable blocks of code. They make your code cleaner and more efficient. Learn how to create and use functions.
<?php
function greet($name) {
return "Hello, " . $name;
}
echo greet("Alice");
?>
Arrays
Arrays store multiple values in one variable. There are different types of arrays: indexed, associative, and multidimensional.
<?php
$fruits = array("Apple", "Banana", "Cherry");
echo $fruits[1]; // Outputs: Banana
?>
Error Handling
Learn how to handle errors. Use try
and catch
blocks to manage exceptions. This keeps your application running smoothly.
<?php
try {
// Code that may throw an exception
} catch (Exception $e) {
echo 'Caught exception: ', $e->getMessage(), "\n";
}
?>
Practice Regularly
The key to mastering PHP is practice. Build small projects. Experiment with different features. Over time, you’ll become more comfortable with PHP.
Resources
Use online resources to learn more. Websites like PHP.net, W3Schools, and Codecademy offer tutorials and documentation.
Conclusion
PHP is a versatile language. With these tips and tricks, you’re on your way to mastering it. Remember to practice regularly and use the resources available to you. Happy coding!
FAQS