PHP is a popular language for web development. It powers many dynamic websites you visit every day. This guide will show you how to use PHP to build dynamic websites.
Setting Up Your Environment
First, you need to set up your PHP environment. Install a local server like XAMPP or MAMP. These tools provide a PHP development environment on your computer.
Basic PHP Syntax
PHP syntax is straightforward. Embed PHP code within HTML using <?php ... ?>
tags. Here’s a simple example:
<!DOCTYPE html>
<html>
<body>
<?php
echo “Hello, World!”;
?>
</body>
</html>
Working with Forms
Forms are essential for interactive websites. PHP makes it easy to handle form data. Use $_POST
to collect data from a form submitted with the POST method.
<!DOCTYPE html>
<html>
<body>
<form method=”post” action=”<?php echo $_SERVER[‘PHP_SELF’];?>”>
Name: <input type=”text” name=”name”>
<input type=”submit”>
</form>
<?php
if ($_SERVER[“REQUEST_METHOD”] == “POST”) {
$name = $_POST[‘name’];
echo “Hello, $name”;
}
?>
</body>
</html>
Connecting to a Database
Dynamic websites often use databases to store and retrieve data. PHP supports various databases, including MySQL. Here’s how to connect to a MySQL database:
<?php
$servername = “localhost”;
$username = “username”;
$password = “password”;
$dbname = “myDB”;
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die(“Connection failed: ” . $conn->connect_error);
}
echo “Connected successfully”;
?>
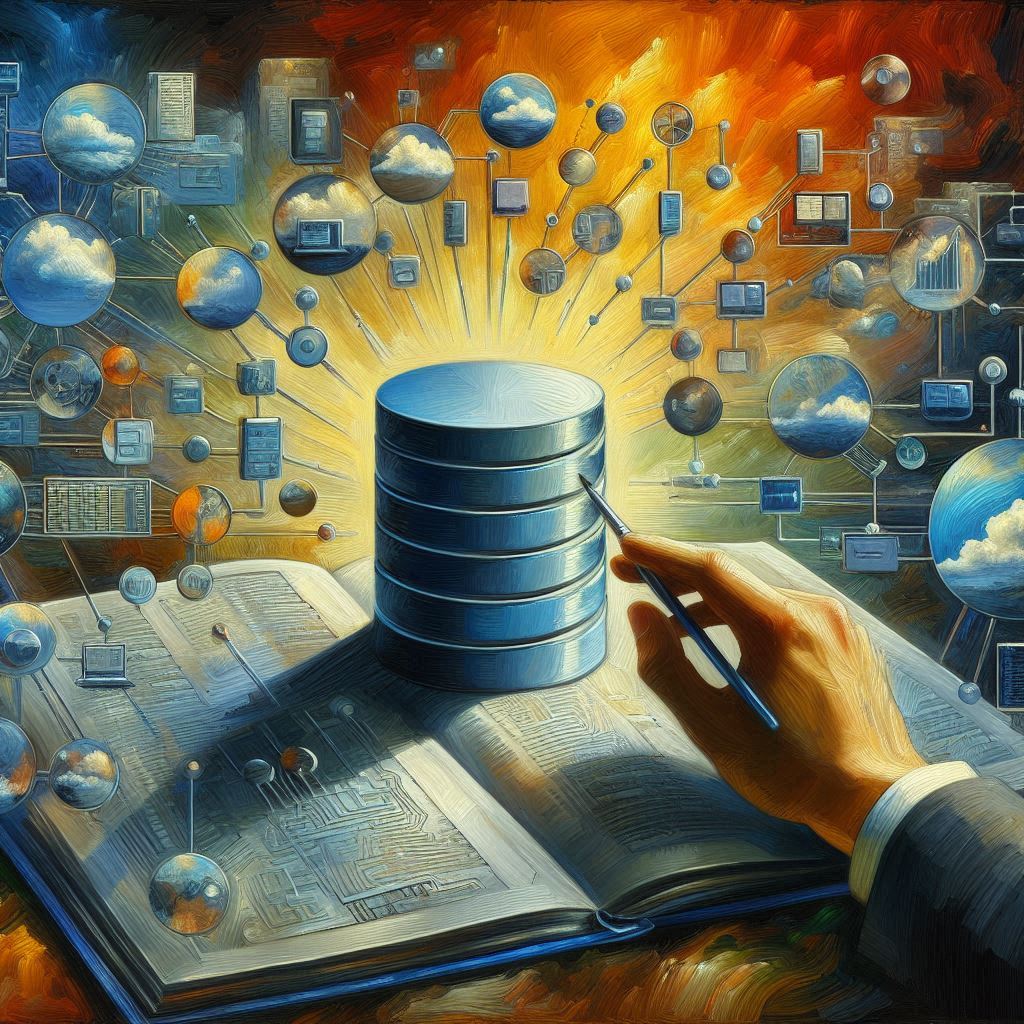
CRUD Operations
CRUD stands for Create, Read, Update, and Delete. These operations are the foundation of dynamic websites.
Create:
$sql = “INSERT INTO MyGuests (firstname, lastname, email)
VALUES (‘John’, ‘Doe’, ‘john@example.com’)”;
if ($conn->query($sql) === TRUE) {
echo “New record created successfully”;
} else {
echo “Error: ” . $sql . “<br>” . $conn->error;
}
Read:
$sql = “SELECT id, firstname, lastname FROM MyGuests”;
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo “id: ” . $row[“id”]. ” – Name: ” . $row[“firstname”]. ” ” . $row[“lastname”]. “<br>”;
}
} else {
echo “0 results”;
}
Update:
$sql = “UPDATE MyGuests SET lastname=’Doe’ WHERE id=2”;
if ($conn->query($sql) === TRUE) {
echo “Record updated successfully”;
} else {
echo “Error updating record: ” . $conn->error;
}
Delete:
$sql = “DELETE FROM MyGuests WHERE id=3”;
if ($conn->query($sql) === TRUE) {
echo “Record deleted successfully”;
} else {
echo “Error deleting record: ” . $conn->error;
}
Using Sessions
Sessions store user data across multiple pages. This is useful for keeping users logged in.
<?php
session_start();
$_SESSION[“username”] = “JohnDoe”;
echo “Session variables are set.”;
?>
Conclusion
Building dynamic websites with PHP is both fun and powerful. By setting up your environment, mastering basic syntax, working with forms, connecting to databases, performing CRUD operations, and using sessions, you can create interactive and dynamic web applications. Keep practicing and exploring more features to become proficient in PHP. Happy coding!
FAQS
Mastering PHP: Essential Tips and Trick
Certainly! Here’s an external link that provides additional resources and tutorials on building dynamic websites with PHP:
Site Point – PHP – Site Point offers a comprehensive collection of PHP tutorials, articles, and guides to help you master building dynamic websites with PHP.